ESP8266 hookup
Expanding on the Garduino project, I wanted to setup a web app that I could use to monitor the current state of my garden. I decided to purchase an ESP8266 WIFI module to accomplish the task of sending requests through my WIFI network. I haven’t yet hooked it up to the system, but I have been writing a simple library for the module that I can use to make simple HTTP GET requests.
To power the WIFI module, you need to use a 3.3v power supply. Here is a schematic:
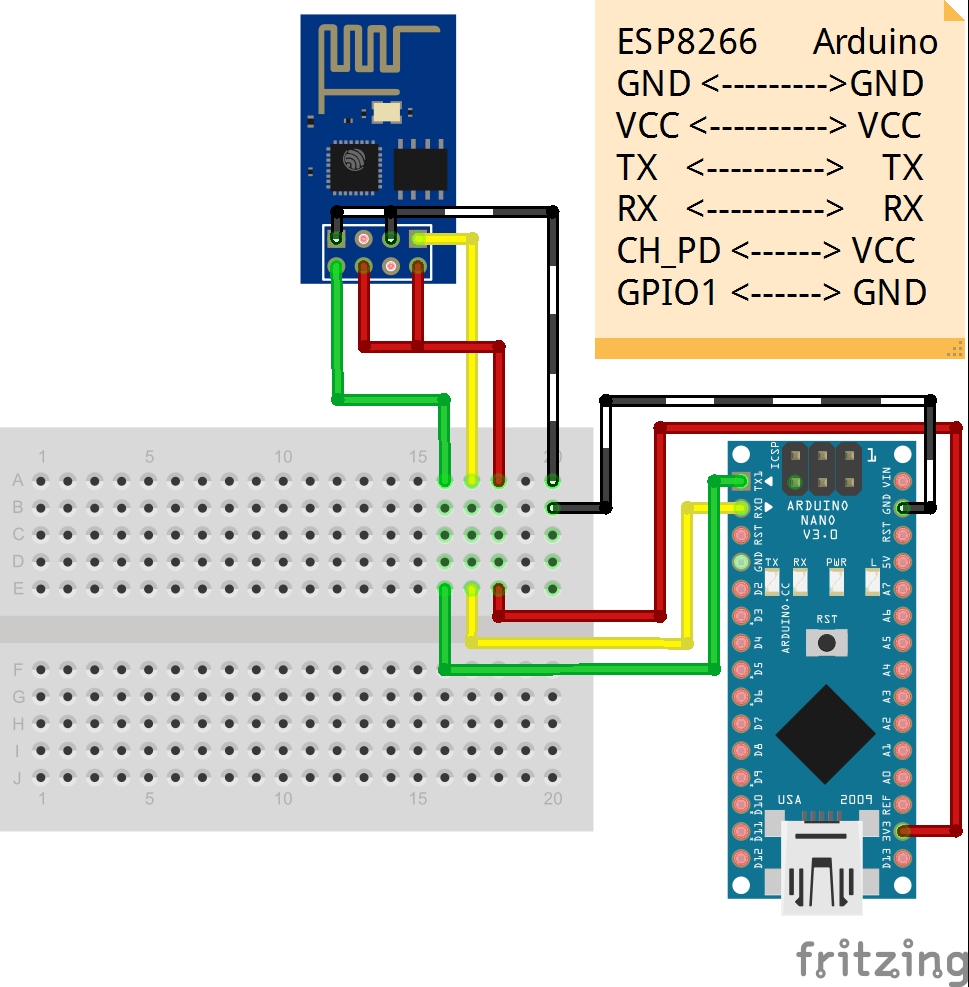
ESP8266 schematic
I used a logic level shifter so that I could hook everything up to my Arduino using the serial ports.
Once everything was wired correctly, I uploaded the following simple sketch:
#include <SoftwareSerial.h>
SoftwareSerial mySerial(10, 11);
void setup() {
// put your setup code here, to run once:
Serial.begin(115200);
mySerial.begin(115200);
}
void loop() {
if (mySerial.available()) {
Serial.write(mySerial.read());
}
if (Serial.available()) {
mySerial.write(Serial.read());
}
}
Because I am using an Uno, I need to use SoftwareSerial to give myself extra serial ports. If you are using another Arduino with multiple serial ports, you can just use the hardware serial. Note that the baud rates match up on both the serial ports. Once this sketch is uploaded, you can fire up the serial monitor from the Arduino IDE.
You can issue commands that will be passed to the ESP8266, and the response will be output into the serial monitor. To test that everything is working correctly, try issuing an:
AT
In the serial monitor (make sure you change the line ending to be a carriage return – \r\n – before sending the command), you should see an:
OK
response. You can find a command reference here. Note that some of these commands may not be available depending on the firmware installed on your specific ESP8266 module. The first thing I did was:
AT+CIOBAUD=9600
This changes the baud rate on the ESP8266 to 9600. Arduino’s SoftwareSerial library has trouble keeping up at 115200 (which was the default baud rate of the ESP8266 that I purchased) so by decreasing it, I solved the issue of garbled output in the serial monitor. Make sure that you change the baud rates in the code to match the baud rate that is set in the ESP8266 (in this case, change the 115200 to 9600 in the above code).
I’m getting ready to hook this up to the Garduino in the next couple days – updates to follow…